This document is an introduction to fractals. It shows how, with the simple concept of recursivity, complex figures can be drawn very easily. The construction of three figures is detailed here: the "dragon", the "snowflake" and the "wave". It is illustrated by the applet written in Java, for which the source code is available.
The construction of fractals is based on recursivity. If you look at a fractal at different scales, you will find the same motif. It is this motif that is the base of the fractal. Indeed, to build a fractal, we consider a very simple form. In the case of the dragon, this is the following motif.
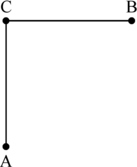
ABC forms an isosceles triangle right-angled in C, C being at the left of the vector AB. To draw the dragon, we use the following recursivity.
To draw a dragon from the point A to the point B, a dragon must be drawn from the point C to the point A and another must be drawn from the point C to the point B, C being such as the triangle ABC is isosceles and right-angled in C and such as C is at the left of the vector AB.
Let us try to draw a dragon from this recursivity. We choose two points A and B.
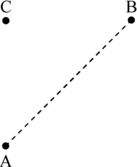
Draw a dragon from A to B comes to draw a dragon from C to A and another one from C to B.
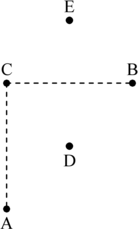
Draw a dragon from C to A comes to draw a dragon from D to C and another one from D to A. In the same way, draw a dragon from C to B comes to draw a dragon from E to C and another one from E to B.
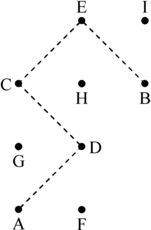
Draw a dragon from D to C comes to draw a dragon from G to D and another one from G to C. In the same way, draw a dragon from D to A comes to... We could go on indefinitely with the recursivity. The first thing to do when drawing a fractal is to fix the depth of the recursivity, meaning the number of times the recursivity is applied. Here, we will stop with three, which corresponds to the moment we need to draw the following dragons: F to A, F to D, G to D, G to C, H to C, H to E, I to E, I to B. And instead of continuing to apply the recursivity, we decide that a dragon is drawn simply as a line and we obtain the following fractal of depth 3.
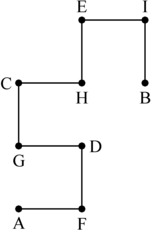
The fractals as we usually know them are simply drawn with a more important depth. Draw a fractal may seem complicated when the depth is high, but in fact, it is very simple to program. Indeed, here is the function that allows the drawing of the dragon fractal from the point A to the point B with a depth p.
function drawDragon(A,B,p):
if (p = 0) then
drawLine(A,B);
else
C ← /* Computing of C */;
drawDragon(C,A,p - 1);
drawDragon(C,B,p - 1);
end if;
end function;
Now we must determine the coordinates (xc;yc) of the point C. We recall that ABC is an isosceles triangle right-angled in C.
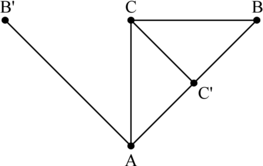
From the figure, the triangle AC'C is also isosceles right-angled in C'. If we consider the vector AB' as perpendicular to AB and with the same length, we can then calculate C the following way.
C ← A + AB / 2 + AB' / 2 |
|
⇔ |
|
xc ← xa + (xb - xa) / 2 - (yb - ya) / 2
yc ← ya + (yb - ya) / 2 + (xb - xa) / 2 |
Here is the dragon fractal at depth 12.
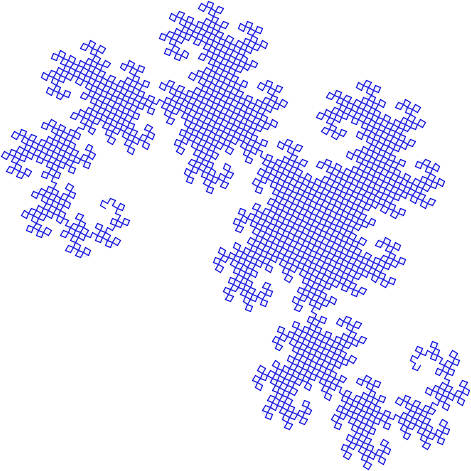
The principle of construction of this fractal is the same as the one of the dragon fractal. We consider the following motif.
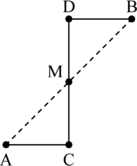
The point M is the middle of the segment [AB], its coordinates (xm;ym) are expressed:
M ← (A + B) / 2 |
|
⇔ |
|
xm ← (xa + xb) / 2
ym ← (ya + yb) / 2 |
The triangle AMC is isosceles right-angled in C and the triangle MDB is isosceles right-angled in D. C is at the right and D is at the left of AB. The coordinates of C and D are computed using the formula established to calculate the point C of the dragon fractal.
Here is the function that allows the drawing of the wave fractal from A to B with a depth p.
function drawWave(A,B,p):
if (p = 0) then
drawLine(A,B);
else
C ← /* Computing of C */;
D ← /* Computing of D */;
drawWave(A,C,p - 1);
drawWave(C,D,p - 1);
drawWave(D,B,p - 1);
end if;
end function;
Here is the wave fractal at depth 11.
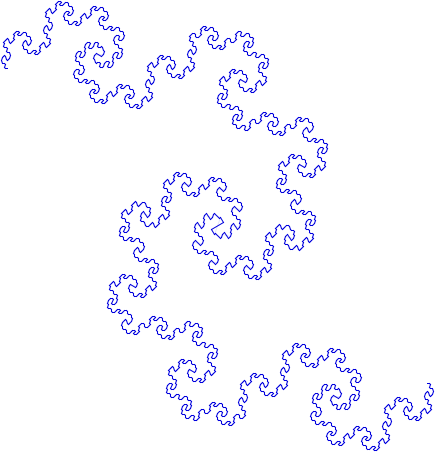
The construction of this fractal is based on the following motif.
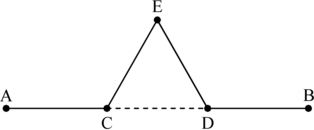
C of coordinates (xc;yc) is on the straight line (AB) at the third of the distance ||AB|| from A. In the same way, D of coordinates (xd;yd) is on the straight line (AB) at the third of the distance ||AB|| of B. Hence, their coordinates are calculated:
C ← A + AB / 3
D ← B - AB / 3 |
|
⇔ |
|
xc ← xa + (xb - xa) / 3
yc ← ya + (yb - ya) / 3
xd ← xb - (xb - xa) / 3
yd ← yb - (yb - ya) / 3 |
E is such as CED forms an equilateral triangle.
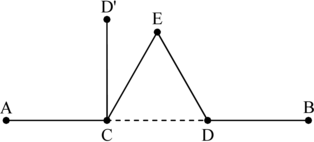
If we consider the vector CD' as perpendicular to CD and with the same length, we can then calculate the coordinates (xe;ye) of E the following way.
E ← |
|
C + CD cos(60) |
|
|
+ CD' sin(60) |
|
|
⇔ |
|
xe ← xc + (xd - xc) / 2 - (yd - yc) √3 / 2
ye ← yc + (yd - yc) / 2 + (xd - xc) √3 / 2 |
Here is the function that allows the drawing of the snowflake fractal from A to B with a depth p.
function drawSnowflake(A,B,p):
if (p = 0) then
drawLine(A,B);
else
C ← /* Computing of C */;
D ← /* Computing of D */;
E ← /* Computing of E */;
drawSnowflake(A,C,p - 1);
drawSnowflake(C,E,p - 1);
drawSnowflake(E,D,p - 1);
drawSnowflake(D,B,p - 1);
end if;
end function;
To draw the snowflake entirely, we must call three times the drawing of the fractal. To do so, we choose three points X, Y and Z that form an equilateral triangle.
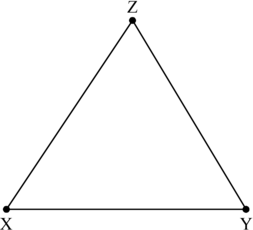
Then fractals are drawn from X to Y, from Y to Z and from Z to X with the same depth.
drawSnowflake(X,Z,p);
drawSnowflake(Z,Y,p);
drawSnowflake(Y,X,p);
Here is the snowflake fractal at depth 4.
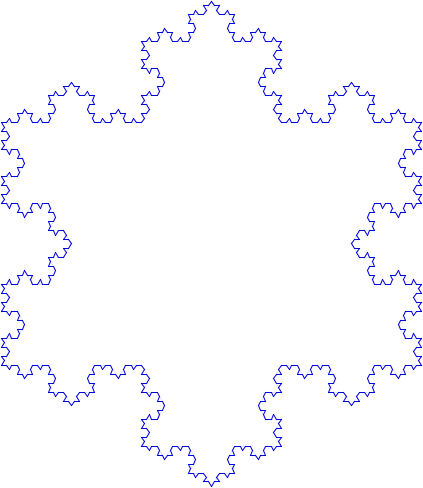
|